Building a Multilingual Website with Next.js 15 and Sanity: A Complete Guide
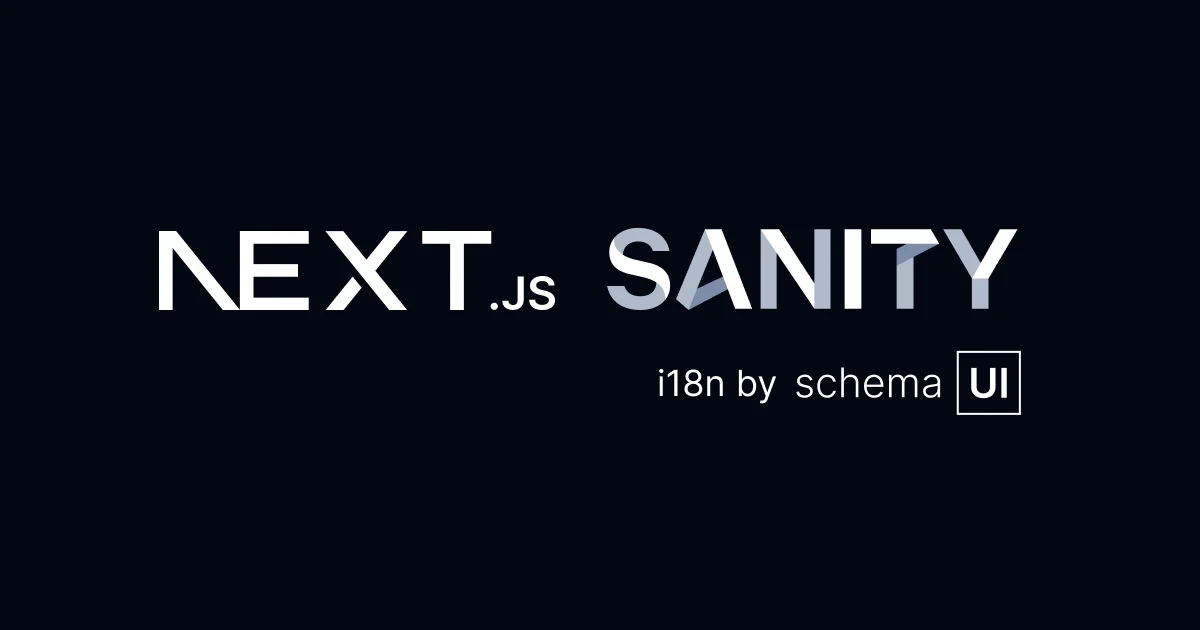
In today's global digital landscape, creating multilingual websites is crucial for reaching a wider audience. This guide will show you how to implement a robust internationalization (i18n) system using Next.js 15 and Sanity CMS, complete with AI-assisted translations.
This implementation is based on the recently featured Schema UI Next.js Sanity Starter template, which provides a powerful page builder with Live Updates and On-Demand Revalidation powered by Sanity Live. The template delivers exceptional performance with 99+ Lighthouse scores on mobile out of the box, and all pages are pre-built using Static Site Generation (SSG).
This guide focuses specifically on the Sanity CMS integration aspects of internationalization. For the Next.js routing implementation, I'm using the official Next.js App Router i18n example, which provides a robust foundation for handling language-based routing and middleware.
Real-World Implementation Results
I recently implemented this internationalization setup for a client project with two locales. The results were impressive:
- Full production deployment with 630 statically generated pages takes only 1 minute and 40 seconds
- The implementation generated significant interest in the developer community after posting on X thread
- Many developers were curious about the setup, which inspired this comprehensive guide
Template Features
- Next.js 15 with App Router
- Sanity v3 with Live Preview by Default
- On-Demand Revalidation with Sanity Live
- Static Site Generation for optimal performance
- Tailwind CSS and shadcn/ui for styling
- Production-ready React components with matching Sanity schemas
Table of Contents
- Setting Up Sanity with i18n Support
- Configuring Document-Level Translations
- Implementing Field-Level Translations
- AI-Assisted Content Translation
- Querying Localized Content
Setting Up Sanity with i18n Support
First, we need to install the necessary Sanity packages for internationalization:
pnpm i @sanity/document-internationalization sanity-plugin-internationalized-array @sanity/assist
Then, update your Sanity configuration to include the internationalization plugins:
import { documentInternationalization } from "@sanity/document-internationalization";import { internationalizedArray } from "sanity-plugin-internationalized-array";import { assist } from "@sanity/assist";export default defineConfig({// ... other configplugins: [// ... other pluginsdocumentInternationalization({supportedLanguages: [{ id: "en", title: "English" },{ id: "es", title: "Spanish" },{ id: "fr", title: "French" },],schemaTypes: ["page", "post", "testimonial", "faq"],}),internationalizedArray({languages: [{ id: "en", title: "English" },{ id: "es", title: "Spanish" },{ id: "fr", title: "French" },],defaultLanguages: ["en"],fieldTypes: ["string"],buttonLocations: ["field"],}),],});
Configuring Document-Level Translations
For document-level translations, Sanity creates separate documents for each language. This approach is perfect for pages, blog posts, and other content types that need complete translation:
export default {name: 'page',title: 'Page',type: 'document',fields: [{name: 'language',type: 'string',readOnly: true,hidden: true,},// ... other fields],};
Implementing Field-Level Translations
For smaller content pieces like category names or metadata, we can use field-level translations:
export default {name: 'category',title: 'Category',type: 'document',fields: [{name: 'title',type: 'internationalizedArray',options: {languages: [{ id: "en", title: "English" },{ id: "es", title: "Spanish" },{ id: "fr", title: "French" },],},},],};
AI-Assisted Content Translation
Sanity's AI Assist feature can help with content translation. Configure it in your Sanity config:
assist({translate: {document: {languageField: "language",documentTypes: ["page", "post", "testimonial", "faq"],},field: {documentTypes: ["category"],languages: [{ id: "en", title: "English" },{ id: "es", title: "Spanish" },{ id: "fr", title: "French" },],},},}),
Querying Localized Content
When fetching content, include the language parameter in your GROQ queries:
export const PAGE_QUERY = groq`*[_type == "page" && slug.current == $slug && language == $language][0]{blocks[]{// ... block queries},meta_title,meta_description,// ... other fields}`;export const PAGES_SLUGS_QUERY = groq`*[_type == "page" && defined(slug) && language == $language]{slug}`;
Benefits of This Setup
The combination of Next.js 15, Sanity v3, and this internationalization approach offers several key advantages:
Lightning-Fast Builds:
- 630 pages generated in just 100 seconds during real world scenario on one of client projects
- Efficient static generation with optimal caching
- Perfect for sites with hundreds of translated pages
Superior Performance:
- 99+ Lighthouse scores on mobile
- Static generation for all pages
- Minimal client-side JavaScript
Content Management Excellence
- Sanity Live by default
- AI-assisted translations
- Field and document-level translation support
- Intuitive content editing experience
Developer Experience:
- Based on production-tested template
- Clean separation of concerns
- Type-safe queries and schemas
- Minimal setup required
Scalability:
- Handles hundreds of pages efficiently
- Easy to add new languages
- Optimized for large content sets
- Production-ready from the start
Short Video Overview with Demo
Conclusion
By following this guide, you've learned how to
- Set up Sanity with internationalization support
- Implement document-level translations for pages and posts
- Add field-level translations for smaller content pieces
- Utilize AI-assisted translation features
- Query localized content effectively
Resources
- Complete Implementation
- Schema UI i18n Example - Full implementation with sample data and 3 languages
- Next.js i18n Example - Official Next.js routing implementation, handles routing and middleware. Used as a foundation for the routing setup. - Documentation
- Sanity Documentation
- Next.js Documentation
- Schema UI Template
This solution provides a flexible and maintainable way to manage multilingual content in your Next.js application while leveraging Sanity's powerful content management capabilities. The combination of Schema UI starter template and Next.js App Router i18n example gives you a production-ready foundation that can handle hundreds of pages efficiently while maintaining excellent performance.